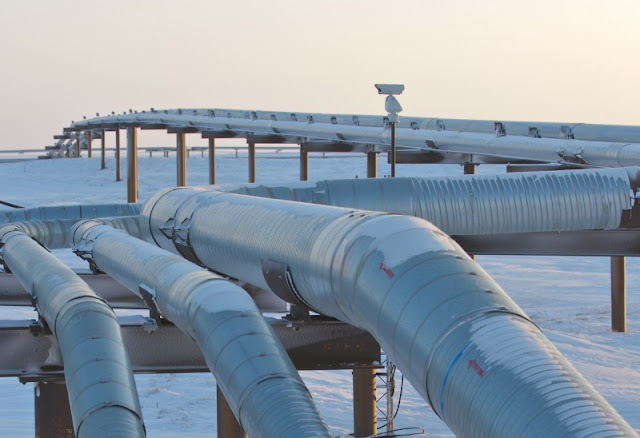
Creating CI/CD Pipeline for Deploying into the kubernetes using Jenkins.
Create the Pipeline script for the Following purpose.
1. Checkout the Code from Git.
2. Build it using the Maven.
3. Create the Docker Image.
4. Push it to the Docker Hub.
5. Deploy the Docker Image to the Kubernetes.
I am using Minikube and docker for the desktop in Windows 10 Machine.
1. Install the Plugin Kubernetes Continuous Deploy.
2. Configure the KubeConfig from Dashboard.
Navigate to Manage Jenkins > Manage Credentials > Jenkins > Global Credentials > Add Credentails
In-Kind Select the Kubernetes Configuration and Define the location from the Kube config.
C:\Users\Syed\.kube\config Then Save it.
3. Make Sure the YAML File is available in the location provided.
4.Create the Pipeline with the following syntax.
pipeline {
environment {
registry = "syedghouse14/greet-user-repo"
registryCredential = 'Docker-Hub'
dockerImage = ''
dockerfile="${workspace}\\GreetUser\\Dockerfile"
pomfile="${workspace}\\GreetUser\\pom.xml"
JAR_FILE="target/*.jar"
}
agent any
stages {
stage('Cloning Git') {
steps {
git 'https://github.com/Syed-SearchEndeca/gretuser.git'
}
}
stage ('Build') {
steps {
withMaven(maven : 'apache-maven-3.6.3') {
bat "mvn clean package -f ${pomfile}"
}
}
}
stage('Building image') {
steps{
script {
dockerImage = docker.build(registry + ":$BUILD_NUMBER",
"--file ${dockerfile} --build-arg JAR_FILE=target/*.jar .")
}
}
}
stage('Deploy Image') {
steps{
script {
docker.withRegistry( '', registryCredential ) {
dockerImage.push()
}
}
}
}
stage('Deploy on kubernetes') {
steps {
script {
kubernetesDeploy(configs: "**/*.yaml", kubeconfigId: "KubeConfig")
}
}
}
}
}